How to Write "Hello, World!" in C Language
Introduction
Learning to code can be both exciting and overwhelming. One of the first programs that every beginner writes is the "Hello, World!" program. It might seem simple, but it's a crucial step in understanding how a programming language works. In this guide, we'll walk through writing this classic program in the C programming language.
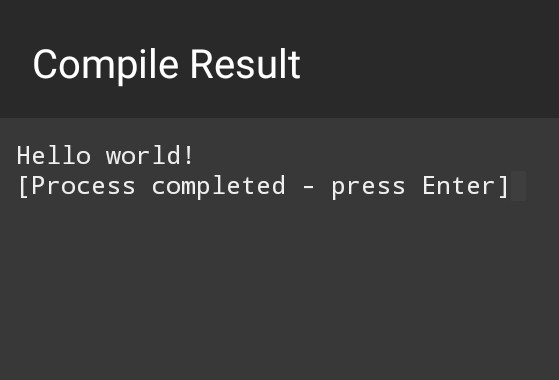
Prerequisites
Before we begin, make sure you have a C compiler installed on your computer. For Windows users, MinGW is a great option. If you are on macOS, you can install Xcode Command Line Tools. For Linux users, GCC is usually pre-installed, but you can install it using your package manager if necessary.
Writing the Code
Open your preferred text editor or IDE, and enter the following code:
#include <stdio.h> int main() { // Print "Hello, World!" to the console printf("Hello, World!\n"); return 0; }
Understanding the Code
Let's break down what each part of the code does:
#include <stdio.h>
: This line tells the compiler to include the standard input-output library, which contains theprintf
function used to output text.int main()
: This defines the main function, which is the starting point of any C program. Every C program must have amain
function.printf("Hello, World!\n");
: This function call outputs the text "Hello, World!" to the console. The\n
is a newline character, which moves the cursor to the next line.return 0;
: This statement ends themain
function and returns 0 to the operating system, signaling that the program executed successfully.
Compiling and Running Your Program
Save the file with a .c
extension, for example, hello.c
. To compile the program, open your terminal or command prompt, navigate to the directory where your file is saved, and run the following command:
gcc hello.c -o hello
This command compiles hello.c
and generates an executable file named hello
. To execute the program, use the command:
./hello
You should see the message Hello, World!
displayed on the console. Congratulations, you've just written and executed your first C program!
Comments in C Code
Comments are an essential part of coding. They help explain what the code does, making it easier for others (and yourself) to understand and maintain. In C, comments are added using //
for single-line comments and /* ... */
for multi-line comments. For instance:
#include <stdio.h> int main() { // This is a single-line comment printf("Hello, World!\n"); /* This is a multi-line comment */ return 0; }
Comments are a great way to document your thought process and make your code more readable. As you write more complex programs, you'll find them invaluable.
Conclusion
Writing a "Hello, World!" program might seem trivial, but it's an important step in learning any programming language. It helps you get familiar with the basic syntax and the process of compiling and running code. Keep experimenting and building on this foundation, and you'll soon be able to tackle more complex projects. Happy coding!